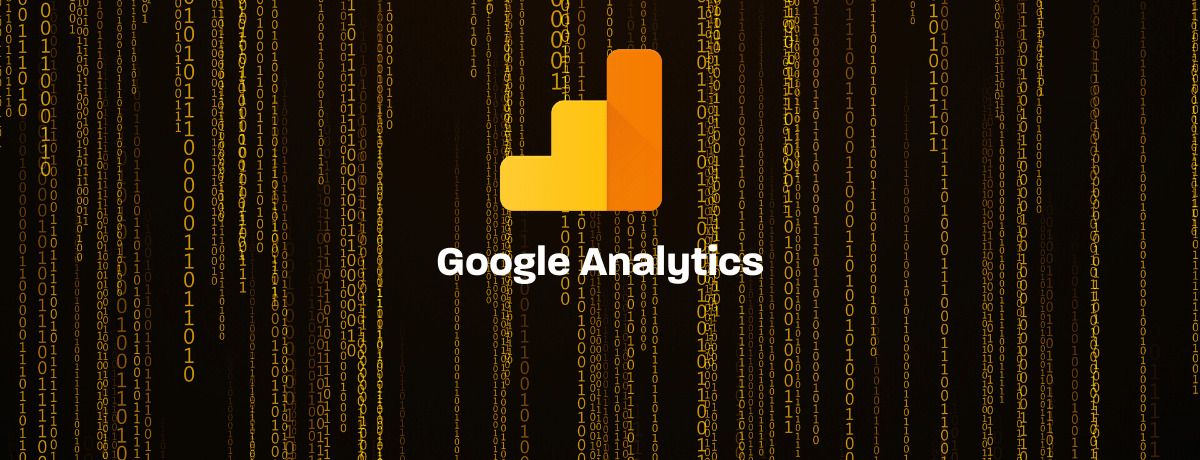
Script to Copy Filters between Google Analytics Accounts
Do you have over 50 filters that you want to replicate and copy from one Google Analytics to another? Unfortunately, Google Analytics doesn’t allow to copy filters between different accounts, copying filters is only available for views within one Google Analytics account. However, I wrote this Google App Script that uses the Google Analytics Management API to read all the filters from a source account and copy them into the new account. The script supports all filter types:
- Exclude Filter
- Include Filter
- Uppercase Filter
- Lowercase Filter
- Search & Replace Filter
- Advanced Filter
How to use the Script?
Navigate to Google App Scripts and create a new project.
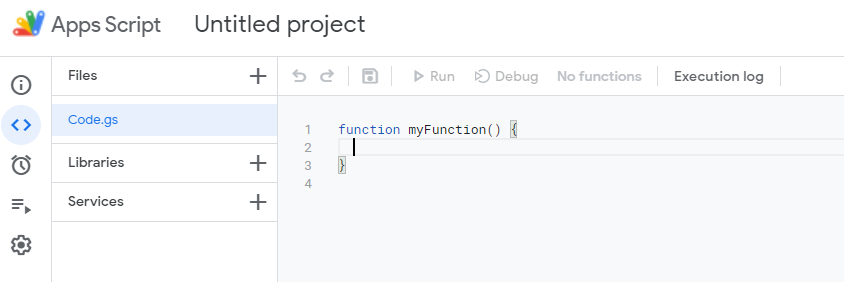
Enable the Google Analytics API by clicking “Services” then adding “Google Analytics API” to your service list.
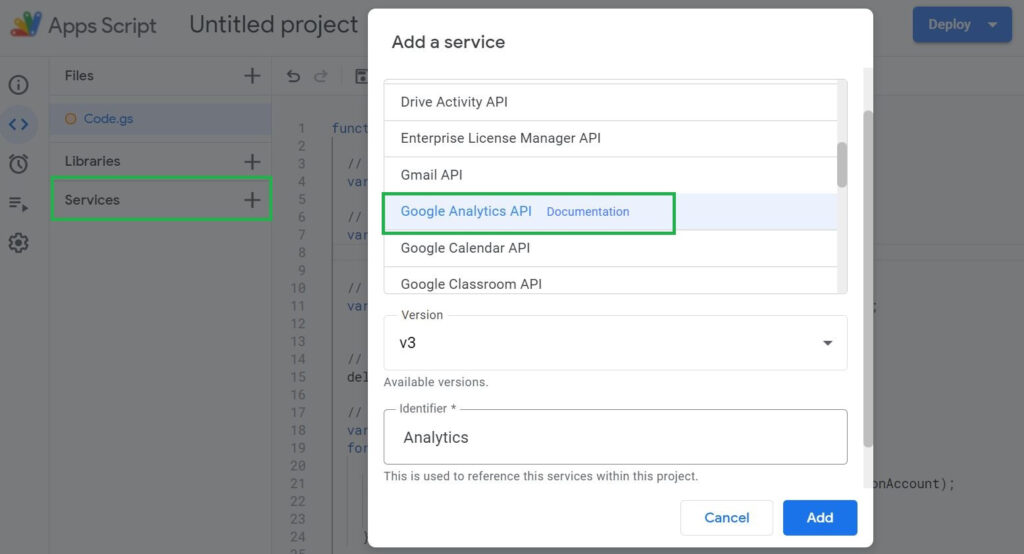
Copy and paste the script below in the code editor:
function myFunction() {
// Source account ID
var sourceAccount = 'xxxxxx';
// Destination account ID
var destinationAccount = 'xxxxxx';
// Pull a list of filters from the source account
var sourceFilters = Analytics.Management.Filters.list(sourceAccount);
// Uncomment if you want to delete all fiters from the destination account
//deleteFilters(destinationAccount);
// Loop through and add the filter name and exclude details
var items = sourceFilters['items'];
for (var i = 0; i<items.length; i++){
results = Analytics.Management.Filters.insert(items[i], destinationAccount);
// Pause for 1 second between adding each filter
Utilities.sleep(1000);
}
}
function deleteFilters(destinationAccount) {
// Pull a list of filters from the destination account
var destinationFilters = Analytics.Management.Filters.list(destinationAccount);
var destionationItems = destinationFilters['items'];
// Loop through and delete every filter ID
for (var i = 0; i<destionationItems.length; i++){
if (destionationItems[i] != undefined){
var filterId = destionationItems[i]['id'];
results = Analytics.Management.Filters.remove(destinationAccount, filterId);
}
// Pause for 1 second between deleting
Utilities.sleep(1000);
}
}
It’s now time to set the source and destination account IDs in the script. You just need to replace ‘xxxxxx’ with your account IDs where sourceAccount is the ID of the Google Analytics account you want to copy filters from, and destinationAccount is the ID of the Google Analytics account you want to copy to.
// Source account ID
var sourceAccount = 'xxxxxx';
// Destination account ID
var destinationAccount = 'xxxxxx';
The script does support deleting all filters from the destination account as well. In order to use this feature, you need to uncomment the delete function by removing the // before deleteFilters(destinationAccount);
// Uncomment if you want to delete all fiters from the destination account
deleteFilters(destinationAccount);
All you need to do now is to run your script and make sure to authorize the script to access your Google Analytics. Please try it out and let me know in the comments if you have any questions.
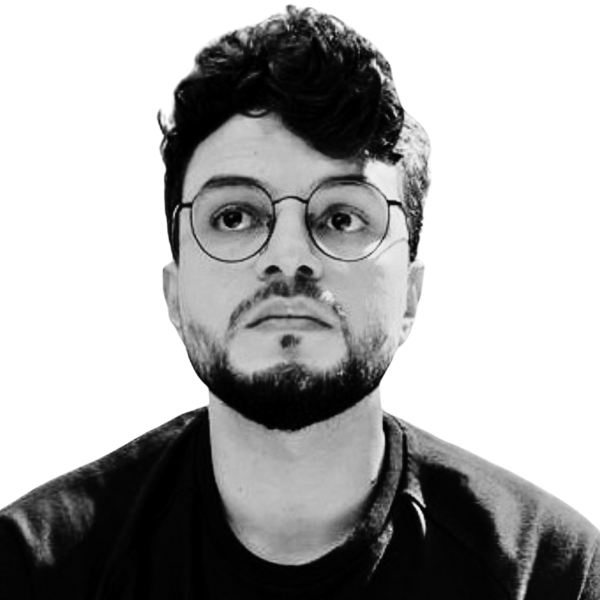
Entrepreneur focused on building MarTech products that bridge the gap between marketing and technology. Check out my latest product GA4 Auditor and discover all my products here.