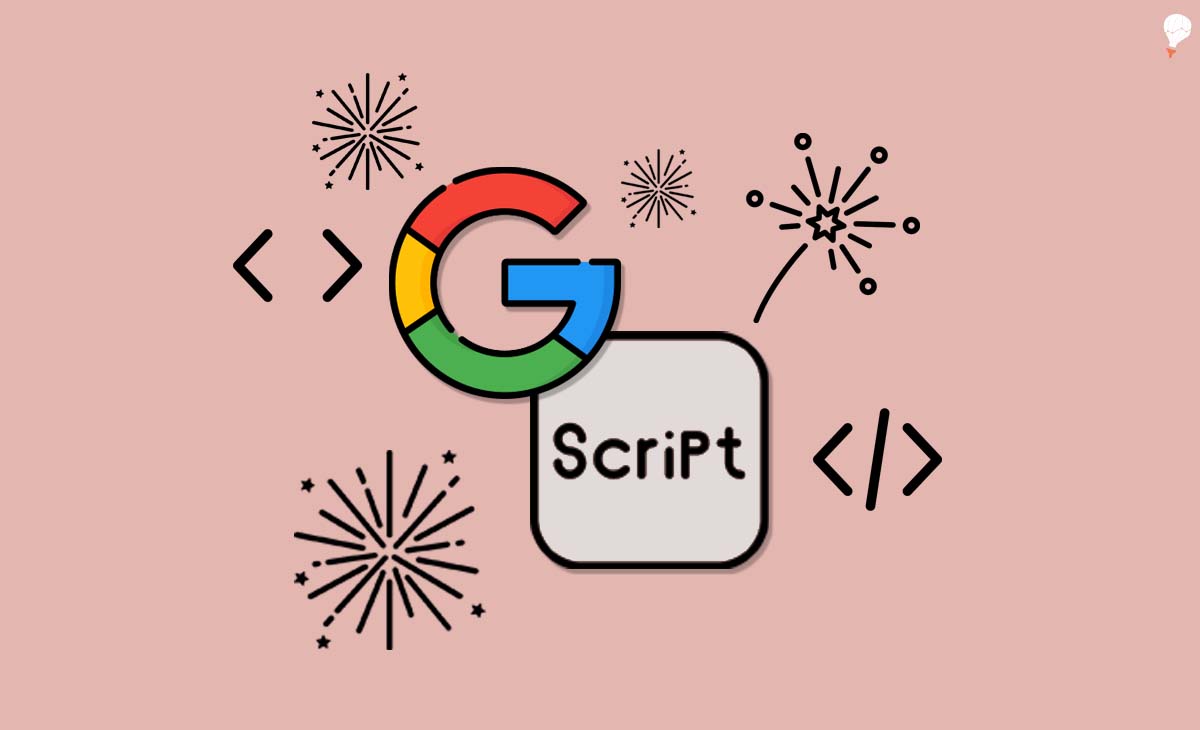
Google Ads Script to Pause/Remove Low Search Volume Keywords
Low search volume is a status that Google Ads gives to keywords with very little to no search history. The keywords remain inactive until the number of search queries for these keywords increases (even a small amount), they’ll be reactivated and will start triggering your ads to show again.
When you have a keyword with low search volume, you have a couple of options:
- Do nothing and wait for the system to automatically check again. If more people start searching for your keyword, Google will reactivate it. This option can be particularly helpful if you’re advertising a new brand, term, or product.
- Pause or Remove these keywords especially if you have a large number of them. This will clutter your account and make it hard to navigate across account entities.
So, if you have a significant number of such keywords, we’ve written a script to help you get rid of these keywords.
How’s the script work?
“Low search volume” status is not currently supported to be called through AdWords script. The workaround here is the script checks campaign, ad group, and keywords with Zero impression for the date range ALL TIME and pauses them respectively. This will make sure that none of your campaigns or ad groups have zero keywords.
What if you recently launched or added new campaigns, ad groups, or keywords, and hence they have zero impressions?
To avoid pausing any new elements you’ve launched and haven’t accrued any data yet, we’ve added a conditional label to the script to prevent it from pausing this new stuff. What you need to do is to create a label for your new campaigns, ad groups, or keywords that the script won’t touch or affect, then modify the label name in the script. In the script source code, you will need to replace NEW with your label name:
var labelName = 'NEW';
The script will pause the keywords by default, in case you want to delete them forever you need to change the value ‘pause’ to ‘remove’:
keyword.pause();// You can replace "pause" with "remove" incase you want to delete them forever.
Here’s the source code for single AdWords accounts;
/*************************************************** * Low Search Volume Keywords Script * Version 1.0 * Created By: Ahmed Ali *https://optimizationup.com/ ****************************************************/ function main() { var labelName = 'NEW'; //add your label here to prevent the script from modifying these items. // Pause Campaigns = 0 Impressions var campaignIterator = AdWordsApp.campaigns() .withCondition("Impressions = 0") .withCondition("Status = ENABLED") .withCondition('LabelNames CONTAINS_NONE ["' + labelName + '"]') .withCondition("AdvertisingChannelType = SEARCH") .forDateRange("ALL_TIME") .get(); while (campaignIterator.hasNext()) { var campaign = campaignIterator.next(); campaign.pause(); } //Pause Ad groups = 0 Impressions var adGroupIterator = AdWordsApp.adGroups() .withCondition("Impressions = 0") .withCondition('CampaignStatus = ENABLED') .withCondition("Status = ENABLED") .withCondition('LabelNames CONTAINS_NONE ["' + labelName + '"]') .withCondition("AdvertisingChannelType = SEARCH") .forDateRange("ALL_TIME") .get(); while (adGroupIterator.hasNext()) { var adGroup = adGroupIterator.next(); adGroup.pause(); } //Pause Keywords = 0 Impressions var keywordsIterator = AdWordsApp.keywords() .withCondition("Impressions = 0") .withCondition('CampaignStatus = ENABLED') .withCondition("AdGroupStatus = ENABLED") .withCondition("Status = ENABLED") .withCondition('LabelNames CONTAINS_NONE ["' + labelName + '"]') .withCondition("AdvertisingChannelType = SEARCH") .forDateRange("ALL_TIME") .get(); while (keywordsIterator.hasNext()) { var keyword = keywordsIterator.next(); keyword.pause(); // You can replace "pause" with "remove" incase you want to delete them forever. } }
Here’s the source code for MCCs:
/*************************************************** * Low Search Volume Keywords Script * Version 1.0 * Created By: Ahmed Ali *https://optimizationup.com/ **************************************************** function main() { var accountIterator = MccApp.accounts().executeInParallel('LowSearchVolume'); } function LowSearchVolume() { var labelName = 'NEW'; // Pause Campaigns = 0 Impressions var campaignIterator = AdWordsApp.campaigns() .withCondition("Impressions = 0") .withCondition("Status = ENABLED") .withCondition('LabelNames CONTAINS_NONE ["' + labelName + '"]') .withCondition("AdvertisingChannelType = SEARCH") .forDateRange("ALL_TIME") .get(); while (campaignIterator.hasNext()) { var campaign = campaignIterator.next(); campaign.pause(); } //Pause Ad groups = 0 Impressions var adGroupIterator = AdWordsApp.adGroups() .withCondition("Impressions = 0") .withCondition('CampaignStatus = ENABLED') .withCondition("Status = ENABLED") .withCondition('LabelNames CONTAINS_NONE ["' + labelName + '"]') .withCondition("AdvertisingChannelType = SEARCH") .forDateRange("ALL_TIME") .get(); while (adGroupIterator.hasNext()) { var adGroup = adGroupIterator.next(); adGroup.pause(); } //Pause Keywords = 0 Impressions var keywordsIterator = AdWordsApp.keywords() .withCondition("Impressions = 0") .withCondition('CampaignStatus = ENABLED') .withCondition("AdGroupStatus = ENABLED") .withCondition("Status = ENABLED") .withCondition('LabelNames CONTAINS_NONE ["' + labelName + '"]') .withCondition("AdvertisingChannelType = SEARCH") .forDateRange("ALL_TIME") .get(); while (keywordsIterator.hasNext()) { var keyword = keywordsIterator.next(); keyword.pause(); // You can replace "pause" with "remove" incase you want to delete them forever. } }
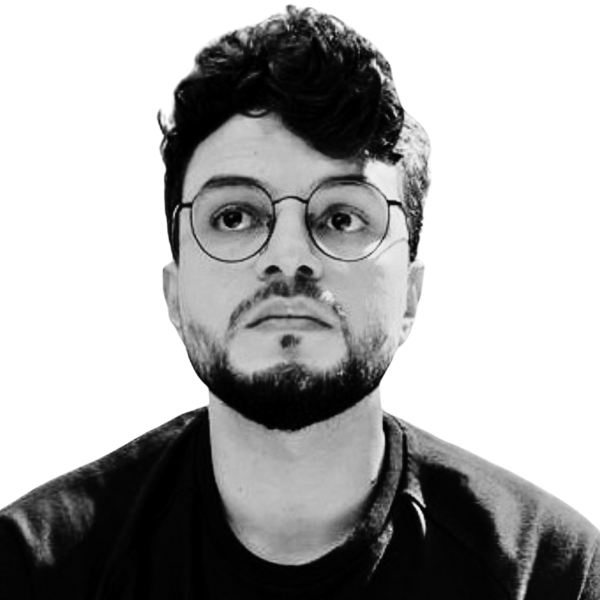
Entrepreneur focused on building MarTech products that bridge the gap between marketing and technology. Check out my latest product GA4 Auditor and discover all my products here.
Currently, you can use SystemServingStatus = ‘RARELY_SERVED’ to get the low search volume keywords from the KEYWORDS_PERFORMANCE_REPORT (by using AWQL query).